Components
Accordions
Accordions are used to toggle sections of content.
<!-- standard Accordions example -->
<div class="accordion">
<input type="checkbox" id="accordion-1" name="accordion-checkbox" hidden>
<label class="accordion-header">
<i class="icon icon-arrow-right mr-1"></i>
Title
</label>
<div class="accordion-body">
<!-- Accordions content -->
</div>
</div>
<!-- mutually exclusive Accordions example (with same input names) -->
<div class="accordion">
<input type="radio" id="accordion-1" name="accordion-radio" hidden>
<label class="accordion-header">
Title
</label>
<div class="accordion-body">
<!-- Accordions content -->
</div>
</div>
Alternatively, you can use details
and summary
instead of input
radio or checkbox trick. Add the open
attribute to details
to expand it. Microsoft Edge support is in development.
<!-- details and summary Accordions example -->
<details class="accordion" open>
<summary class="accordion-header">
<i class="icon icon-arrow-right mr-1"></i>
Title
</summary>
<div class="accordion-body">
<!-- Accordions content -->
</div>
</details>
Autocomplete
Autocomplete form component provides suggestions while you type. It is often used for tags and contacts input.
Add a container element with the form-autocomplete
class. There are 2 parts of it, one is form-autocomplete-input
, another is menu
component. You may add the is-focused
class to form-autocomplete-input
to make it appear as focus state.
Spectre.css does NOT include JavaScript code, you will need to implement your JS to interact with the autocomplete. The autocomplete HTML structure is exampled below.
<div class="form-autocomplete">
<!-- autocomplete input container -->
<div class="form-autocomplete-input form-input">
<!-- autocomplete chips -->
<div class="chip">
<img src="img/avatar-1.png" class="avatar avatar-sm" alt="Thor Odinson">
Thor Odinson
<a href="#" class="btn btn-clear" aria-label="Close" role="button"></a>
</div>
<!-- autocomplete real input box -->
<input class="form-input" type="text" placeholder="typing here">
</div>
<!-- autocomplete suggestion list -->
<ul class="menu">
<!-- menu list chips -->
<li class="menu-item">
<a href="#">
<div class="tile tile-centered">
<div class="tile-icon">
<img src="img/avatar-4.png" class="avatar avatar-sm" alt="Steve Rogers">
</div>
<div class="tile-content">
Steve Rogers
</div>
</div>
</a>
</li>
</ul>
</div>
Avatars
Avatars are user profile pictures.
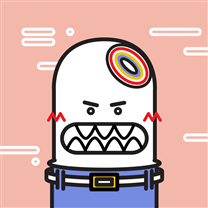
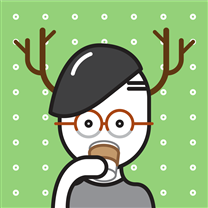
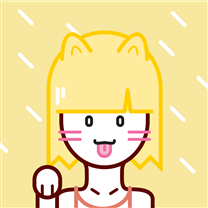
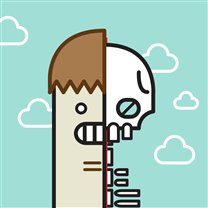
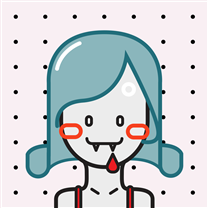
Add the avatar
class to <img> element. There are 4 additional sizes available, including avatar-xl
(64px), avatar-lg
(48px), avatar-sm
(24px), and avatar-xs
(16px). By default, the avatar size is 32px.
For users who don't have profile pictures, you may use their initials for avatars. Add the avatar
class and avatar size class to <div> element. The data-initial
attribute is the name appear inside the avatar.
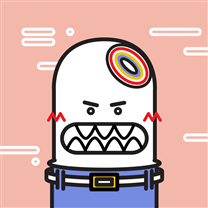
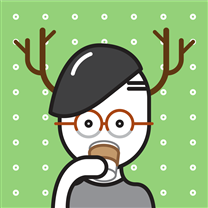
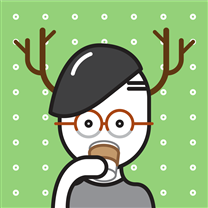
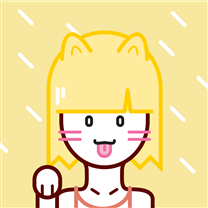
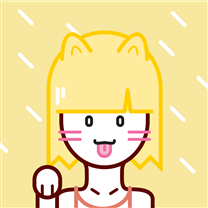
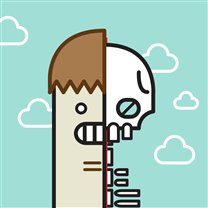
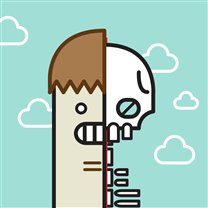
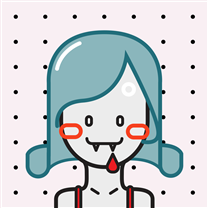
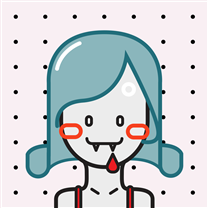
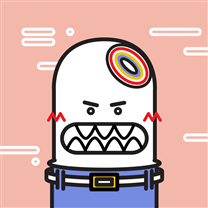
<!-- Basic avatar examples -->
<figure class="avatar avatar-xl">
<img src="img/avatar-1.png" alt="...">
</figure>
<figure class="avatar avatar-xl">
<img src="img/avatar-1.png" alt="...">
<img src="img/avatar-5.png" class="avatar-icon" alt="...">
</figure>
<figure class="avatar avatar-xl" data-initial="YZ" style="background-color: #5755d9;"></figure>
<!-- Show initals when avatar image is unavailable or not fully loaded -->
<figure class="avatar avatar-xl" data-initial="YZ" style="background-color: #5755d9;">
<img src="img/avatar-1.png" alt="...">
</figure>
Avatars support presence indicators. You can add an i
element with the avatar-presence
class, and add online
, busy
or away
class for different status colors. The default is gray which means offline.
<figure class="avatar avatar-xl">
<img src="img/avatar-1.png" alt="...">
<i class="avatar-presence online"></i>
</figure>
Badges
Badges are often used as unread number indicators.
Add the badge
class to non self closing elements. And add the data-badge
attribute to define the content of a badge. The badge will appear in the top-right direction of the element.
If there is no data-badge
or the attribute is not specified, the badge will appear as a dot.
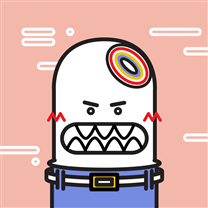
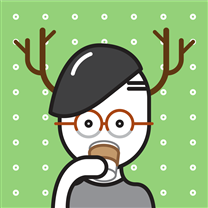
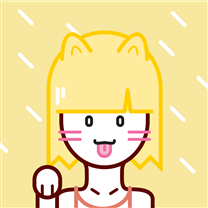
Badges support button
and avatars
elements as well.
<span class="badge">
Notifications
</span>
<span class="badge" data-badge="8">
Notifications
</span>
<button class="btn badge" data-badge="8">
Button
</button>
<figure class="avatar badge" data-badge="8" data-initial="YZ">
<img src="img/avatar-3.png" alt="YZ">
</figure>
Bars
Bars represent the progress of a task or the value within the known range. Bars are custom components for displaying HTML5 progress
, meter
and input range
elements.
Add a container element with the bar
class. And add child elements with the bar-item
class. The width percentage value is needed for every bar-item
.
There is the bar-sm
class for thinner Bars. Also, you could use Tooltips for any bar-item
.
<div class="bar bar-sm">
<div class="bar-item" role="progressbar" style="width:25%;" aria-valuenow="25" aria-valuemin="0" aria-valuemax="100"></div>
</div>
<!-- multi-bars -->
<div class="bar">
<div class="bar-item tooltip" data-tooltip="25%" style="width:25%;">25%</div>
<div class="bar-item" style="width:15%;background:#818bd5;">15%</div>
</div>
Slider bars
You can add the bar-slider
class to the Bars container. And add child elements with the bar-item
class and bar-slider-btn
inside bar-item. You need to set the bar-item
width manually to have the slider point.
If there are two bar-item
divs in one bar-slider, you will have a range slider.
<!-- slider -->
<div class="bar bar-slider">
<div class="bar-item" role="progressbar" style="width:25%;">
<button class="bar-slider-btn btn" role="slider"></button>
</div>
</div>
<!-- range slider -->
<div class="bar bar-slider">
<div class="bar-item" role="progressbar" style="width:15%;">
<button class="bar-slider-btn btn" role="slider"></button>
</div>
<div class="bar-item" role="progressbar" style="width:65%;">
<button class="bar-slider-btn btn" role="slider"></button>
</div>
</div>
Cards
Cards are flexible content containers.
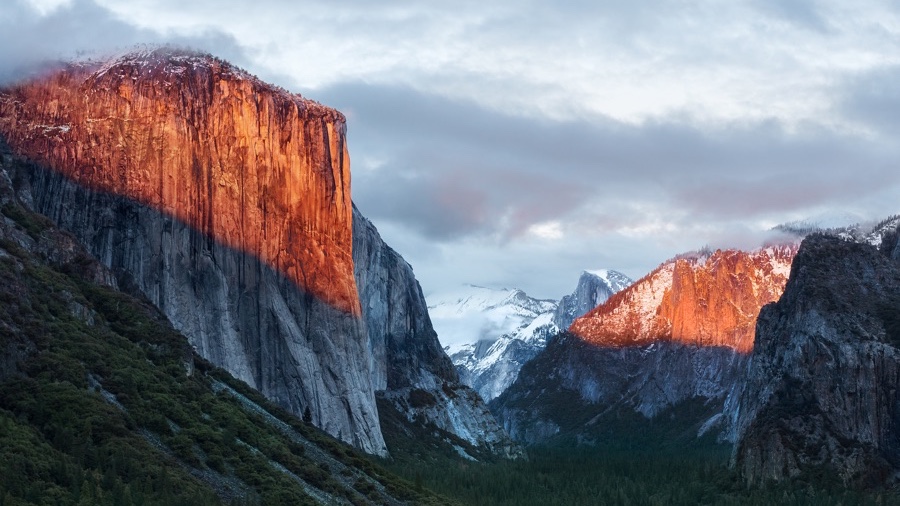
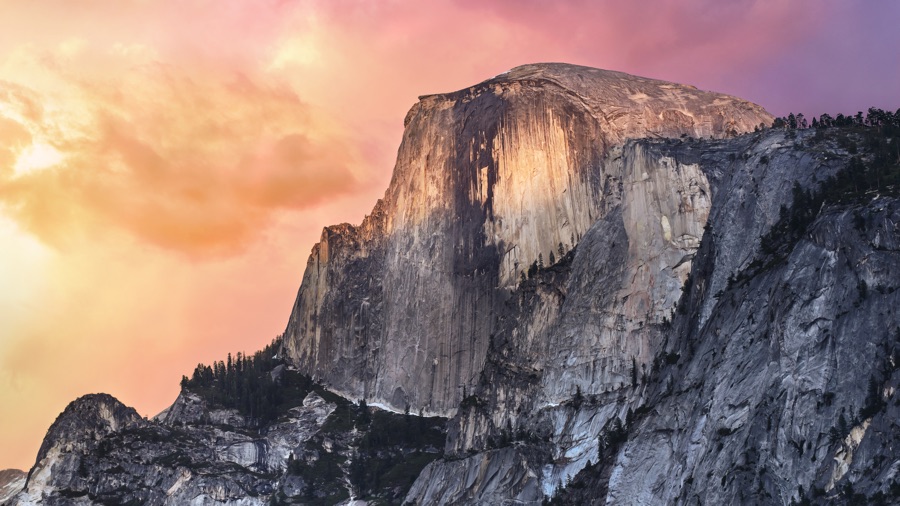
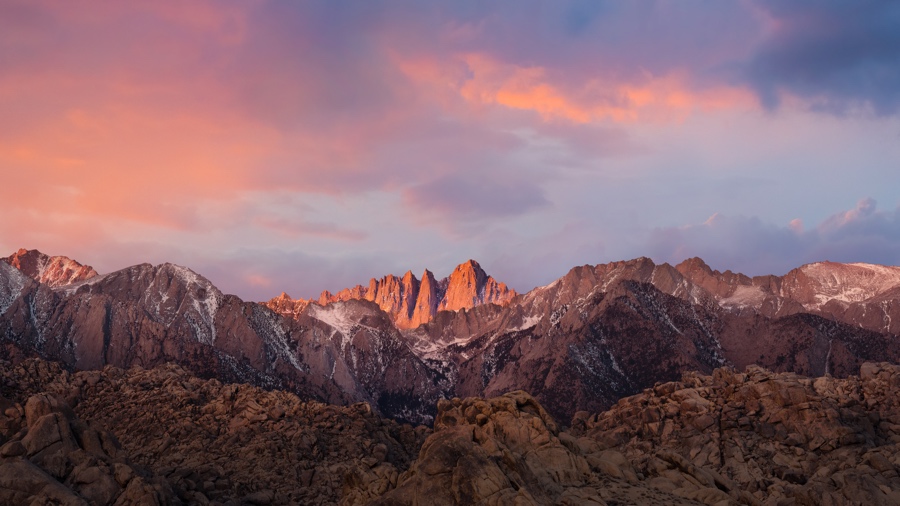
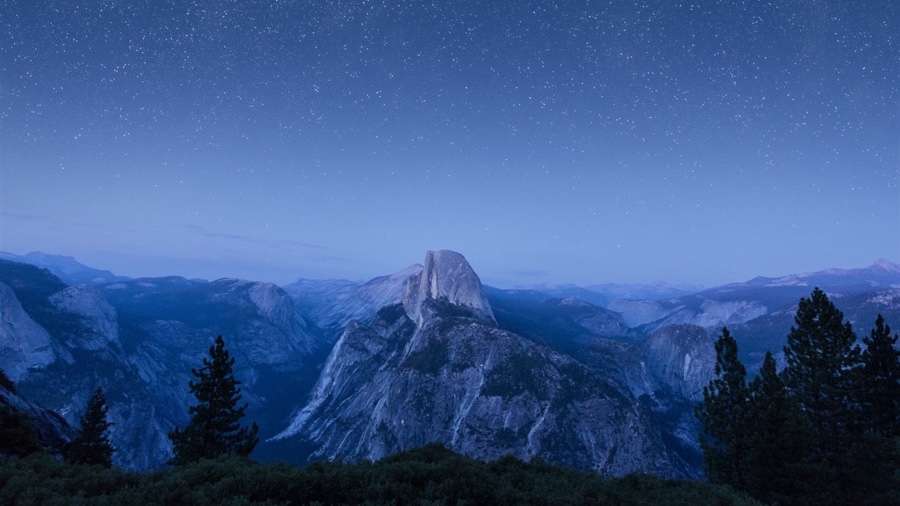
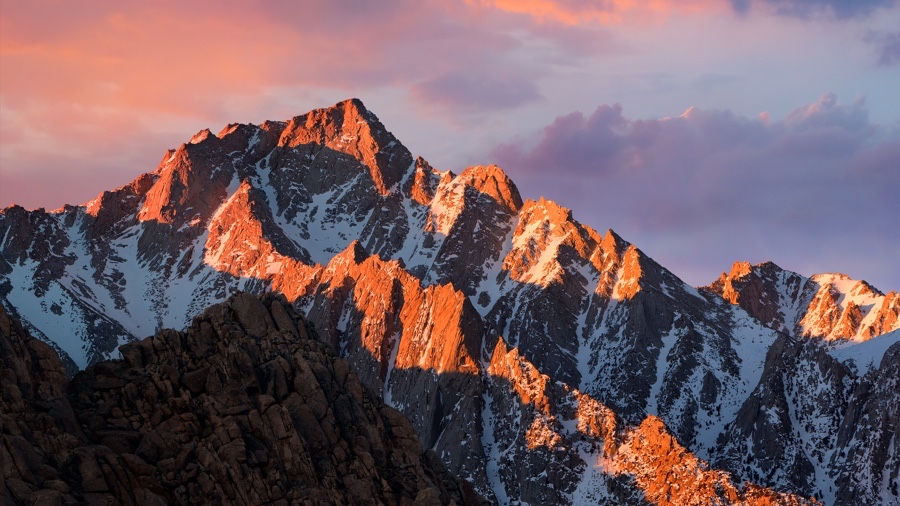
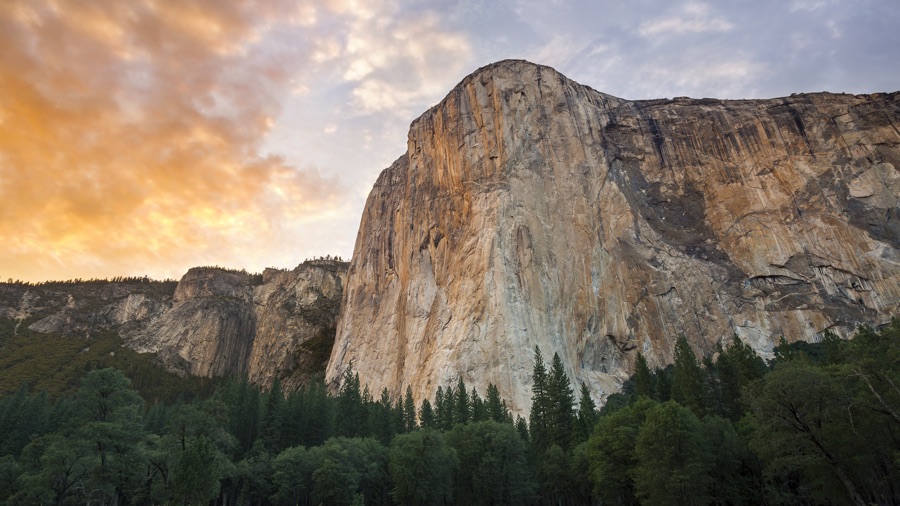
Add a container element with the card
class. And add child elements with the card-image
, card-header
, card-body
and/or card-footer
classes. The card-image
can be placed in any position.
<div class="card">
<div class="card-image">
<img src="img/osx-el-capitan.jpg" class="img-responsive">
</div>
<div class="card-header">
<div class="card-title h5">Microsoft</div>
<div class="card-subtitle text-gray">Software and hardware</div>
</div>
<div class="card-body">
Empower every person and every organization on the planet to achieve more.
</div>
<div class="card-footer">
<button class="btn btn-primary">Do</button>
</div>
</div>
Chips
Chips are complex entities in small blocks.
Add a container element with the chip
class. And add child text element, buttons or avatars with the avatar
class.
<span class="chip">Crime</span>
<span class="chip">
Biography
<a href="#" class="btn btn-clear" aria-label="Close" role="button"></a>
</span>
<div class="chip">
<img src="img/avatar-1.png" class="avatar avatar-sm">
Yan Zhu
<a href="#" class="btn btn-clear" aria-label="Close" role="button"></a>
</div>
Empty states
Empty states/blank slates are commonly used as placeholders for first time use, empty data and error screens.
You have no new messages
Click the button to start a conversation
You've successfully signed up
Click the button to invite your friends
You are not following anyone
Start to meet new friends
An empty state component can include icons, messages (title and subtitle messages) and action buttons or any combination of those elements. Add empty-icon
, empty-title
, empty-subtitle
or empty-action
to the elements. HTML structure is exampled below.
<div class="empty">
<div class="empty-icon">
<i class="icon icon-people"></i>
</div>
<p class="empty-title h5">You have no new messages</p>
<p class="empty-subtitle">Click the button to start a conversation.</p>
<div class="empty-action">
<button class="btn btn-primary">Send a message</button>
</div>
</div>
Modals
Modals are flexible dialog prompts.
This is the content inside the modal.
Lorem ipsum
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Praesent risus leo, dictum in vehicula sit amet, feugiat tempus tellus. Duis quis sodales risus. Etiam euismod ornare consequat.
Climb leg rub face on everything give attitude nap all day for under the bed. Chase mice attack feet but rub face on everything hopped up on goofballs.
Cupcake ipsum
Jelly-o sesame snaps halvah croissant oat cake cookie. Cheesecake bear claw topping. Chupa chups apple pie carrot cake chocolate cake caramels.
De braaaiiiins apocalypsi gorger omero prefrontal cortex undead survivor fornix dictum mauris. Hi brains mindless mortuis limbic cortex soulless creaturas optic nerve.
Candy ipsum
Efficiently unleash cross-media information without cross-media value. Quickly maximize timely deliverables for real-time schemas. Dramatically maintain clicks-and-mortar.
Caerphilly swiss fromage frais. Brie cheese and wine fromage frais chalk and cheese danish fontina smelly cheese who moved my cheese cow.
Add a container element with the modal
class. And add a real container modal-container
and overlay modal-overlay
inside it. You can add child elements with the modal-header
, modal-content
and modal-footer
- any or all of them.
Spectre.css does not include JavaScript code, you will need to implement your JS to interact with modals. To make a modal appear, add the active
class to the modal
container.
<div class="modal active" id="modal-id">
<a href="#close" class="modal-overlay" aria-label="Close"></a>
<div class="modal-container">
<div class="modal-header">
<a href="#close" class="btn btn-clear float-right" aria-label="Close"></a>
<div class="modal-title h5">Modal title</div>
</div>
<div class="modal-body">
<div class="content">
<!-- content here -->
</div>
</div>
<div class="modal-footer">
...
</div>
</div>
</div>
Modal sizes
Use the modal-sm
class for a smaller modal dialog. The container max width is 320px
.
This is the content inside the modal.
Lorem ipsum
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Praesent risus leo, dictum in vehicula sit amet, feugiat tempus tellus. Duis quis sodales risus. Etiam euismod ornare consequat.
Climb leg rub face on everything give attitude nap all day for under the bed. Chase mice attack feet but rub face on everything hopped up on goofballs.
Cupcake ipsum
Jelly-o sesame snaps halvah croissant oat cake cookie. Cheesecake bear claw topping. Chupa chups apple pie carrot cake chocolate cake caramels.
De braaaiiiins apocalypsi gorger omero prefrontal cortex undead survivor fornix dictum mauris. Hi brains mindless mortuis limbic cortex soulless creaturas optic nerve.
Candy ipsum
Efficiently unleash cross-media information without cross-media value. Quickly maximize timely deliverables for real-time schemas. Dramatically maintain clicks-and-mortar.
Caerphilly swiss fromage frais. Brie cheese and wine fromage frais chalk and cheese danish fontina smelly cheese who moved my cheese cow.
Use the modal-lg
class for a full size modal. The container max width is 960px
.
<div class="modal modal-sm">
<a href="#close" class="modal-overlay" aria-label="Close"></a>
<div class="modal-container">
<!-- modal structure here -->
</div>
</div>
Pagination
Add a container element with the pagination
class. And add child elements with the page-item
class. The page-item
with the active
class will be highlighted. You can add the disabled
to the page-item
to have unclickable page links.
<ul class="pagination">
<li class="page-item disabled">
<a href="#" tabindex="-1">Previous</a>
</li>
<li class="page-item active">
<a href="#">1</a>
</li>
<li class="page-item">
<a href="#">2</a>
</li>
<li class="page-item">
<a href="#">3</a>
</li>
<li class="page-item">
<span>...</span>
</li>
<li class="page-item">
<a href="#">12</a>
</li>
<li class="page-item">
<a href="#">Next</a>
</li>
</ul>
You could use previous and next pagination to navigate.
<ul class="pagination">
<li class="page-item page-prev">
<a href="#">
<div class="page-item-subtitle">Previous</div>
<div class="page-item-title h5">Getting started</div>
</a>
</li>
<li class="page-item page-next">
<a href="#">
<div class="page-item-subtitle">Next</div>
<div class="page-item-title h5">Layout</div>
</a>
</li>
</ul>
Panels
Panels are flexible view container with auto-expand content section.
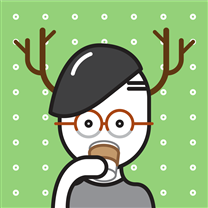
Thor Odinson
Earth's Mightiest Heroes joined forces to take on threats that were too big for any one hero to tackle...
Bruce Banner
The Strategic Homeland Intervention, Enforcement, and Logistics Division...
Tony Stark
Earth's Mightiest Heroes joined forces to take on threats that were too big for any one hero to tackle...
Steve Rogers
The Strategic Homeland Intervention, Enforcement, and Logistics Division...
Natasha Romanoff
Earth's Mightiest Heroes joined forces to take on threats that were too big for any one hero to tackle...
Add a container element with the panel
class. And add child elements with the panel-header
, panel-nav
, panel-body
and/or panel-footer
class. The panel-body
can be auto expanded and vertically scrollable.
<div class="panel">
<div class="panel-header">
<div class="panel-title">Comments</div>
</div>
<div class="panel-nav">
<!-- navigation components: tabs, breadcrumbs or pagination -->
</div>
<div class="panel-body">
<!-- contents -->
</div>
<div class="panel-footer">
<!-- buttons or inputs -->
</div>
</div>
Popovers
Popovers are small overlay content containers. Popovers component is built entirely in CSS.
Wrap an element by a container with the popover
class. And add a container with the popover-container
next to the element. You can use Cards component inside the popover-container
.
Also, you can add the popover-right
, popover-bottom
or popover-left
class to define the position. By default, the popovers appear above the element.
<div class="popover popover-right">
<button class="btn btn-primary">right popover</button>
<div class="popover-container">
<div class="card">
<div class="card-header">
...
</div>
<div class="card-body">
...
</div>
<div class="card-footer">
...
</div>
</div>
</div>
</div>
Steps
Steps are progress indicators of a sequence of task steps.
Add a container element with the step
class. And add child elements with the step-item
class. The step-item
with the active
class will be highlighted and indicate the current state of progress.
<ul class="step">
<li class="step-item">
<a href="#" class="tooltip" data-tooltip="Step 1">Step 1</a>
</li>
<li class="step-item active">
<a href="#" class="tooltip" data-tooltip="Step 2">Step 2</a>
</li>
<li class="step-item">
<a href="#" class="tooltip" data-tooltip="Step 3">Step 3</a>
</li>
<li class="step-item">
<a href="#" class="tooltip" data-tooltip="Step 4">Step 4</a>
</li>
</ul>
Tabs
Tabs enable quick switch between different views.
Add a container element with the tab
class. And add child elements with the tab-item
class. You can add the tab-block
class for a full-width tab. The tab-item
or its child <a> with the active
class will be highlighted.
<ul class="tab tab-block">
<li class="tab-item active">
<a href="#">Music</a>
</li>
<li class="tab-item">
<a href="#" class="active">Playlists</a>
</li>
<li class="tab-item">
<a href="#">Radio</a>
</li>
<li class="tab-item">
<a href="#">Connect</a>
</li>
</ul>
If you need badges
on tabs, you can add badge class to the element within tab-item
.
<ul class="tab tab-block">
<li class="tab-item active">
<a href="#" class="badge" data-badge="9">
Music
</a>
</li>
</ul>
You could add a search box or buttons inside a tab. Add the tab-action
class to the tab-item
.
<ul class="tab">
<li class="tab-item active">
<a href="#">
Music
</a>
</li>
<li class="tab-item tab-action">
<div class="input-group input-inline">
<input class="form-input input-sm" type="text" placeholder="search">
<button class="btn btn-primary btn-sm input-group-btn">Search</button>
</div>
</li>
</ul>
Tiles
Tiles are repeatable or embeddable information blocks.
The Avengers
Earth's Mightiest Heroes joined forces to take on threats that were too big for any one hero to tackle...
The S.H.I.E.L.D.
The Strategic Homeland Intervention, Enforcement, and Logistics Division...
Add a container with the tile
class. And add child elements with the tile-icon
, tile-content
or/and tile-action
classes. The tile-icon
and tile-action
are optional.
There are tile-title
and tile-subtitle
classes for title and subtitle text styles.
<div class="tile">
<div class="tile-icon">
<div class="example-tile-icon">
<i class="icon icon-file centered"></i>
</div>
</div>
<div class="tile-content">
<p class="tile-title">The Avengers</p>
<p class="tile-subtitle text-gray">Earth's Mightiest Heroes joined forces to take on threats that were too big for any one hero to tackle...</p>
</div>
<div class="tile-action">
<button class="btn btn-primary">Join</button>
</div>
</div>
Compact tiles
There is compact version of Tiles component, which is often used as contact and file info blocks.
Add the tile-centered
class to the container tile
. The tile-icon
, tile-content
and tile-action
will be vertically centered.
<div class="tile tile-centered">
<div class="tile-icon">
<div class="example-tile-icon">
<i class="icon icon-file centered"></i>
</div>
</div>
<div class="tile-content">
<div class="tile-title">spectre-docs.pdf</div>
<div class="tile-subtitle text-gray">14MB · Public · 1 Jan, 2017</div>
</div>
<div class="tile-action">
<button class="btn btn-link">
<i class="icon icon-more-vert"></i>
</button>
</div>
</div>
Toasts
Toasts are used to show alert or information to users.
Toast Title
Lorem ipsum dolor sit amet, consectetur adipiscing elit.Add a container element with the toast
class. You can add any text within the container, and even icons. You may also add a close button with the btn-clear
class if you need.
And you can add the toast-primary
, toast-success
, toast-warning
or toast-error
class for additional toast colors.
<div class="toast">
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
</div>
<div class="toast toast-primary">
<button class="btn btn-clear float-right"></button>
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
</div>
Tooltips
Tooltips provide context information labels that appear on hover and focus.
Tooltips component is built entirely in CSS.
Add the tooltip
class and the data-tooltip
attribute, which contains the tooltip content, to non self closing elements. And add the tooltip-right
, tooltip-bottom
or tooltip-left
class to define the position of a tooltip. By default, the tooltip appears above the element.
<button class="btn tooltip" data-tooltip="Lorem ipsum dolor sit amet">top tooltip</button>
<button class="btn tooltip tooltip-right" data-tooltip="Lorem ipsum dolor sit amet">right tooltip</button>